기능
점수 기능, 맵 제작(배경, 바닥)

- 수정한 csv 내용
맵은 일단 대충 제작했는데 왜!! 자꾸 오브젝트간 공백이 생기는건지.. 아마 내 생각에는 일러스트에서 가져올때 살짝 삐져나온 부분 제외하고 투명부분이 돼서 그 크기만큼 크기가 특정돼서 공백이 생기는 것 같은데 너무 짜증나서 그냥 오브젝트끼리 재생성될때 바닥은 0.01f만큼 겹치고(1%), 배경은 0.05f(5%)만큼 겹치게 했다.
치즈도 csv파일에서 장애물 생성한 것 처럼 불러와서 썼다.
type이라는 속성을 추가해서 1이면 장애물, 2면 치즈를 불러온다.
치즈를 먹으면 점수를 1점씩 추가한다.
아마 바닥도 csv파일로 변경할 것 같다. 아직은 아니지만....
코드
PlayerController.cs
using System.Collections;
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public Rigidbody2D rb;
public float jumpHeight = 3f;
float highestJumpHeight;
bool grounded = false;
bool invincible = false;
public GameObject normalObject;
public GameObject jumpObject;
public GameObject doubleJumpObject;
public GameObject slideObject;
private bool isSliding = false;
void Start()
{
if (!rb)
{
rb = GetComponent<Rigidbody2D>();
}
}
void Update()
{
bool slideInput = Input.GetKey(KeyCode.DownArrow);
bool jumpInput = Input.GetKeyDown(KeyCode.Space);
if (slideInput && grounded)
{
// 슬라이드 중일 때
isSliding = true;
ActivateSlide();
}
else
{
// 슬라이드 중이 아닐 때
isSliding = false;
DeactivateSlide();
if (grounded)
{
// 땅에 있을 때
OnTheGround(jumpInput);
}
else
{
// 땅에 없을 때
NotOnTheGround(jumpInput);
}
}
}
// 땅에 있을 때의 입력 처리
void OnTheGround(bool jumpInput)
{
normalObject.SetActive(true);
jumpObject.SetActive(false);
doubleJumpObject.SetActive(false);
if (jumpInput)
{
Jump();
}
}
// 땅에 없을 때의 입력 처리
void NotOnTheGround(bool jumpInput)
{
if (jumpObject.activeSelf && jumpInput)
{
DoubleJump();
}
}
// 점프
void Jump()
{
rb.velocity = Vector3.zero;
rb.AddForce(Vector2.up * jumpHeight, ForceMode2D.Impulse);
grounded = false;
jumpObject.SetActive(true);
normalObject.SetActive(false);
doubleJumpObject.SetActive(false);
// 최고 높이 갱신
highestJumpHeight = rb.position.y + jumpHeight;
}
// 더블 점프
void DoubleJump()
{
float doubleJumpForce = jumpHeight;
rb.velocity = Vector3.zero;
rb.AddForce(Vector2.up * doubleJumpForce, ForceMode2D.Impulse);
jumpObject.SetActive(false);
doubleJumpObject.SetActive(true);
normalObject.SetActive(false);
}
// 충돌 감지
private void OnCollisionEnter2D(Collision2D collision)
{
if (collision.gameObject.CompareTag("Obstacle") && !invincible)
{
Debug.Log("충돌!");
StartCoroutine(InvincibleTime());
collision.collider.enabled = false;
}
else if (collision.gameObject.CompareTag("Ground"))
{
grounded = true;
highestJumpHeight = rb.position.y;
}
else if (collision.gameObject.CompareTag("Cheese"))
{
Debug.Log("치즈 냠");
GameManager.Instance.PlayerHitCheese();
Destroy(collision.gameObject); // 충돌한 치즈 삭제
}
}
// 무적상태
IEnumerator InvincibleTime()
{
invincible = true;
yield return new WaitForSeconds(1f);
invincible = false;
}
// 슬라이드 활성화
void ActivateSlide()
{
slideObject.SetActive(true);
normalObject.SetActive(false);
jumpObject.SetActive(false);
doubleJumpObject.SetActive(false);
}
// 슬라이드 비활성화
void DeactivateSlide()
{
slideObject.SetActive(false);
}
}
GameMagager.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class GameManager : MonoBehaviour
{
public static GameManager Instance;
public GameObject cheesePrefab;
public Text scoreTxt;
int totalScore;
private void Awake()
{
Instance = this;
Time.timeScale = 1.0f;
}
void Start()
{
totalScore = 0;
UpdateScoreText();
}
// 플레이어가 치즈와 충돌했을 때 함수
public void PlayerHitCheese()
{
totalScore++;
UpdateScoreText();
}
// 점수 업데이트 함수
void UpdateScoreText()
{
scoreTxt.text = totalScore.ToString();
}
}
ObjectsController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO;
public class ObjectsController : MonoBehaviour
{
private class MapInfo
{
public float mapNum;
public int type;
public float time;
public Vector3 position;
public bool spawned;
}
public GameObject obstaclePrefab;
public GameObject cheesePrefab;
public float speed = 2f;
private List<MapInfo> mapInfos = new List<MapInfo>();
private int currentIndex = 0;
private float gameStartTime;
void Start()
{
gameStartTime = Time.time;
ReadCSV();
}
// CSV파일 읽는 함수
void ReadCSV()
{
string filePath = "Assets/Datas/map_data.csv";
string[] lines = File.ReadAllLines(filePath);
for (int i = 1; i < lines.Length; i++)
{
string line = lines[i];
string[] values = line.Split(',');
float mapNum;
int type;
float time;
float xPosition;
float yPosition;
if (values.Length >= 5 &&
float.TryParse(values[0].Trim(), out mapNum) &&
int.TryParse(values[1].Trim(), out type) &&
float.TryParse(values[2].Trim(), out time) &&
float.TryParse(values[3].Trim(), out xPosition) &&
float.TryParse(values[4].Trim(), out yPosition))
{
float relativeTime = time - gameStartTime;
mapInfos.Add(new MapInfo { mapNum = mapNum, type = type, time = relativeTime, position = new Vector3(xPosition, yPosition, 0), spawned = false });
}
}
StartCoroutine(SpawnObstacles());
}
// 장애물 생성 함수
IEnumerator SpawnObstacles()
{
while (currentIndex < mapInfos.Count)
{
if (mapInfos[currentIndex].time <= Time.time)
{
GameObject prefab;
if (mapInfos[currentIndex].type == 1) // 장애물
prefab = obstaclePrefab;
else if (mapInfos[currentIndex].type == 2) // 치즈
prefab = cheesePrefab;
else
{
Debug.LogError("타입 잘못씀 고치삼");
yield break;
}
if (prefab == null)
{
Debug.LogError("프리팹 오류");
yield break;
}
// 오브젝트 생성
GameObject obstacle = Instantiate(prefab, mapInfos[currentIndex].position, Quaternion.identity);
// 오브젝트 이동
if (mapInfos[currentIndex].type == 1 || mapInfos[currentIndex].type == 2)
{
obstacle.AddComponent<ObstacleMovement>();
obstacle.GetComponent<ObstacleMovement>().speed = speed;
}
mapInfos[currentIndex].spawned = true;
currentIndex++;
}
yield return null;
}
}
}
// 이동 함수
public class ObstacleMovement : MonoBehaviour
{
public float speed = 1f;
void Update()
{
transform.Translate(Vector3.left * speed * Time.deltaTime);
if (transform.position.x < -10f)
{
Destroy(gameObject);
}
}
}
어려웠거나 알게된 점
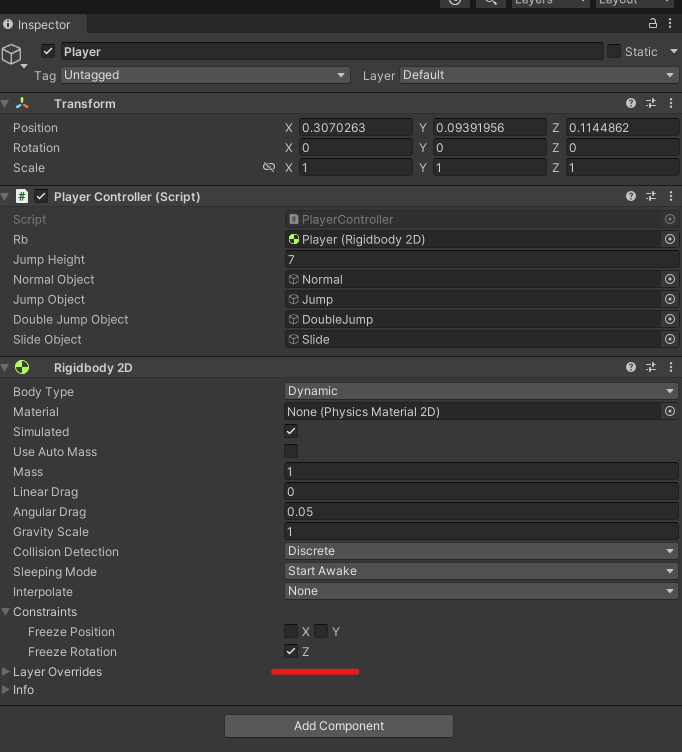
나는 쿠키런을 구현할때 중력만 구현하고 싶었는데 자꾸 좌우로도 넘어지길래 알아봤는데 여기서 이걸 체크하면 중력만 작용하도록 설정할 수 있다.
계획
[ 개발 일정 계획표 ]2024-04-09- 플레이어 점프 기능, 그라운드 스크롤, 배경 스크롤 2024-04-10- 더블 점프 기능, 장애물 설치(csv파일로 연결해서 장애물 생성), 장애물 충돌 이벤트 설정2024-04-11- 로딩화면(스플래시) 제작 및 설정, 전체적인 씬 UI 구현2024-04-12- 기본 좀비맛 스프라이트 이용하여 애니메이션 설정, 쿠키 슬라이드 기능2024-04-13- 점수 기능, 맵 제작(배경, 바닥)
2024-04-14
- 화폐 관리, 뽑기 기능
2024-04-15
- 내 쿠키 확인 기능
'Unity > Project' 카테고리의 다른 글
[Unity] Unity 프로젝트 화면 SNS 공유하기(UnityNativeShare 플러그인) (0) | 2025.02.12 |
---|---|
[Unity] step5-1. 좀비런: 찌끄만 버그들 픽스와 맵 디자인^^ - 계획을 지키지 못한 바보의 일기 (3) | 2024.04.14 |
[Unity] step4. 좀비런: 좀비맛 스프라이트 이용하여 애니메이션 설정, 쿠키 슬라이드 기능(Feat. 내배캠 - TIL) (0) | 2024.04.12 |
[Unity] step3. 좀비런: UI와 로딩화면 제작 (2) | 2024.04.11 |
[Unity] step2. 좀비런: 더블 점프 기능, 장애물 설치 (Feat. 내배캠 - TIL) (2) | 2024.04.10 |
기능
점수 기능, 맵 제작(배경, 바닥)

- 수정한 csv 내용
맵은 일단 대충 제작했는데 왜!! 자꾸 오브젝트간 공백이 생기는건지.. 아마 내 생각에는 일러스트에서 가져올때 살짝 삐져나온 부분 제외하고 투명부분이 돼서 그 크기만큼 크기가 특정돼서 공백이 생기는 것 같은데 너무 짜증나서 그냥 오브젝트끼리 재생성될때 바닥은 0.01f만큼 겹치고(1%), 배경은 0.05f(5%)만큼 겹치게 했다.
치즈도 csv파일에서 장애물 생성한 것 처럼 불러와서 썼다.
type이라는 속성을 추가해서 1이면 장애물, 2면 치즈를 불러온다.
치즈를 먹으면 점수를 1점씩 추가한다.
아마 바닥도 csv파일로 변경할 것 같다. 아직은 아니지만....
코드
PlayerController.cs
using System.Collections;
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public Rigidbody2D rb;
public float jumpHeight = 3f;
float highestJumpHeight;
bool grounded = false;
bool invincible = false;
public GameObject normalObject;
public GameObject jumpObject;
public GameObject doubleJumpObject;
public GameObject slideObject;
private bool isSliding = false;
void Start()
{
if (!rb)
{
rb = GetComponent<Rigidbody2D>();
}
}
void Update()
{
bool slideInput = Input.GetKey(KeyCode.DownArrow);
bool jumpInput = Input.GetKeyDown(KeyCode.Space);
if (slideInput && grounded)
{
// 슬라이드 중일 때
isSliding = true;
ActivateSlide();
}
else
{
// 슬라이드 중이 아닐 때
isSliding = false;
DeactivateSlide();
if (grounded)
{
// 땅에 있을 때
OnTheGround(jumpInput);
}
else
{
// 땅에 없을 때
NotOnTheGround(jumpInput);
}
}
}
// 땅에 있을 때의 입력 처리
void OnTheGround(bool jumpInput)
{
normalObject.SetActive(true);
jumpObject.SetActive(false);
doubleJumpObject.SetActive(false);
if (jumpInput)
{
Jump();
}
}
// 땅에 없을 때의 입력 처리
void NotOnTheGround(bool jumpInput)
{
if (jumpObject.activeSelf && jumpInput)
{
DoubleJump();
}
}
// 점프
void Jump()
{
rb.velocity = Vector3.zero;
rb.AddForce(Vector2.up * jumpHeight, ForceMode2D.Impulse);
grounded = false;
jumpObject.SetActive(true);
normalObject.SetActive(false);
doubleJumpObject.SetActive(false);
// 최고 높이 갱신
highestJumpHeight = rb.position.y + jumpHeight;
}
// 더블 점프
void DoubleJump()
{
float doubleJumpForce = jumpHeight;
rb.velocity = Vector3.zero;
rb.AddForce(Vector2.up * doubleJumpForce, ForceMode2D.Impulse);
jumpObject.SetActive(false);
doubleJumpObject.SetActive(true);
normalObject.SetActive(false);
}
// 충돌 감지
private void OnCollisionEnter2D(Collision2D collision)
{
if (collision.gameObject.CompareTag("Obstacle") && !invincible)
{
Debug.Log("충돌!");
StartCoroutine(InvincibleTime());
collision.collider.enabled = false;
}
else if (collision.gameObject.CompareTag("Ground"))
{
grounded = true;
highestJumpHeight = rb.position.y;
}
else if (collision.gameObject.CompareTag("Cheese"))
{
Debug.Log("치즈 냠");
GameManager.Instance.PlayerHitCheese();
Destroy(collision.gameObject); // 충돌한 치즈 삭제
}
}
// 무적상태
IEnumerator InvincibleTime()
{
invincible = true;
yield return new WaitForSeconds(1f);
invincible = false;
}
// 슬라이드 활성화
void ActivateSlide()
{
slideObject.SetActive(true);
normalObject.SetActive(false);
jumpObject.SetActive(false);
doubleJumpObject.SetActive(false);
}
// 슬라이드 비활성화
void DeactivateSlide()
{
slideObject.SetActive(false);
}
}
GameMagager.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class GameManager : MonoBehaviour
{
public static GameManager Instance;
public GameObject cheesePrefab;
public Text scoreTxt;
int totalScore;
private void Awake()
{
Instance = this;
Time.timeScale = 1.0f;
}
void Start()
{
totalScore = 0;
UpdateScoreText();
}
// 플레이어가 치즈와 충돌했을 때 함수
public void PlayerHitCheese()
{
totalScore++;
UpdateScoreText();
}
// 점수 업데이트 함수
void UpdateScoreText()
{
scoreTxt.text = totalScore.ToString();
}
}
ObjectsController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO;
public class ObjectsController : MonoBehaviour
{
private class MapInfo
{
public float mapNum;
public int type;
public float time;
public Vector3 position;
public bool spawned;
}
public GameObject obstaclePrefab;
public GameObject cheesePrefab;
public float speed = 2f;
private List<MapInfo> mapInfos = new List<MapInfo>();
private int currentIndex = 0;
private float gameStartTime;
void Start()
{
gameStartTime = Time.time;
ReadCSV();
}
// CSV파일 읽는 함수
void ReadCSV()
{
string filePath = "Assets/Datas/map_data.csv";
string[] lines = File.ReadAllLines(filePath);
for (int i = 1; i < lines.Length; i++)
{
string line = lines[i];
string[] values = line.Split(',');
float mapNum;
int type;
float time;
float xPosition;
float yPosition;
if (values.Length >= 5 &&
float.TryParse(values[0].Trim(), out mapNum) &&
int.TryParse(values[1].Trim(), out type) &&
float.TryParse(values[2].Trim(), out time) &&
float.TryParse(values[3].Trim(), out xPosition) &&
float.TryParse(values[4].Trim(), out yPosition))
{
float relativeTime = time - gameStartTime;
mapInfos.Add(new MapInfo { mapNum = mapNum, type = type, time = relativeTime, position = new Vector3(xPosition, yPosition, 0), spawned = false });
}
}
StartCoroutine(SpawnObstacles());
}
// 장애물 생성 함수
IEnumerator SpawnObstacles()
{
while (currentIndex < mapInfos.Count)
{
if (mapInfos[currentIndex].time <= Time.time)
{
GameObject prefab;
if (mapInfos[currentIndex].type == 1) // 장애물
prefab = obstaclePrefab;
else if (mapInfos[currentIndex].type == 2) // 치즈
prefab = cheesePrefab;
else
{
Debug.LogError("타입 잘못씀 고치삼");
yield break;
}
if (prefab == null)
{
Debug.LogError("프리팹 오류");
yield break;
}
// 오브젝트 생성
GameObject obstacle = Instantiate(prefab, mapInfos[currentIndex].position, Quaternion.identity);
// 오브젝트 이동
if (mapInfos[currentIndex].type == 1 || mapInfos[currentIndex].type == 2)
{
obstacle.AddComponent<ObstacleMovement>();
obstacle.GetComponent<ObstacleMovement>().speed = speed;
}
mapInfos[currentIndex].spawned = true;
currentIndex++;
}
yield return null;
}
}
}
// 이동 함수
public class ObstacleMovement : MonoBehaviour
{
public float speed = 1f;
void Update()
{
transform.Translate(Vector3.left * speed * Time.deltaTime);
if (transform.position.x < -10f)
{
Destroy(gameObject);
}
}
}
어려웠거나 알게된 점
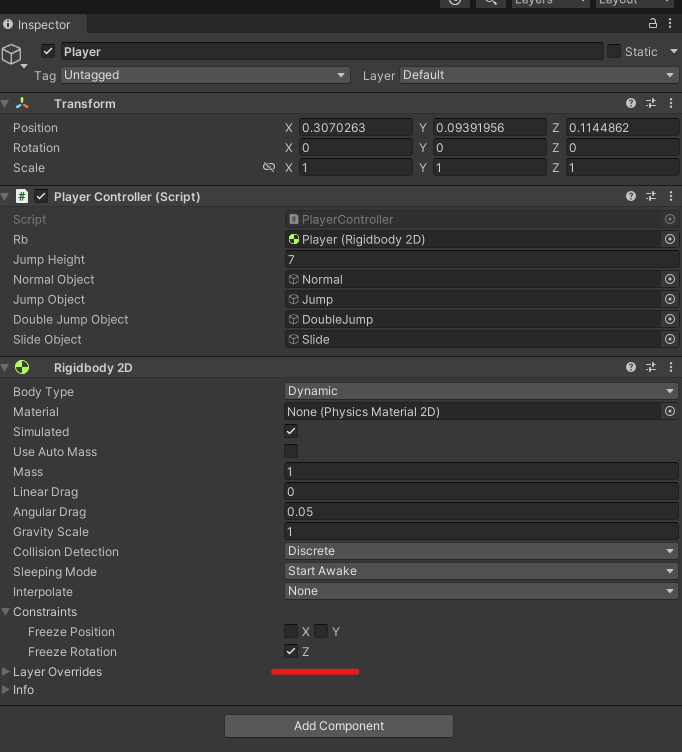
나는 쿠키런을 구현할때 중력만 구현하고 싶었는데 자꾸 좌우로도 넘어지길래 알아봤는데 여기서 이걸 체크하면 중력만 작용하도록 설정할 수 있다.
계획
[ 개발 일정 계획표 ]2024-04-09- 플레이어 점프 기능, 그라운드 스크롤, 배경 스크롤 2024-04-10- 더블 점프 기능, 장애물 설치(csv파일로 연결해서 장애물 생성), 장애물 충돌 이벤트 설정2024-04-11- 로딩화면(스플래시) 제작 및 설정, 전체적인 씬 UI 구현2024-04-12- 기본 좀비맛 스프라이트 이용하여 애니메이션 설정, 쿠키 슬라이드 기능2024-04-13- 점수 기능, 맵 제작(배경, 바닥)
2024-04-14
- 화폐 관리, 뽑기 기능
2024-04-15
- 내 쿠키 확인 기능
'Unity > Project' 카테고리의 다른 글
[Unity] Unity 프로젝트 화면 SNS 공유하기(UnityNativeShare 플러그인) (0) | 2025.02.12 |
---|---|
[Unity] step5-1. 좀비런: 찌끄만 버그들 픽스와 맵 디자인^^ - 계획을 지키지 못한 바보의 일기 (3) | 2024.04.14 |
[Unity] step4. 좀비런: 좀비맛 스프라이트 이용하여 애니메이션 설정, 쿠키 슬라이드 기능(Feat. 내배캠 - TIL) (0) | 2024.04.12 |
[Unity] step3. 좀비런: UI와 로딩화면 제작 (2) | 2024.04.11 |
[Unity] step2. 좀비런: 더블 점프 기능, 장애물 설치 (Feat. 내배캠 - TIL) (2) | 2024.04.10 |